Aug
1
Written by:
Michael Washington
8/1/2011 9:37 AM
Note: If you are new to LightSwitch, it is suggested that you start here: Online Ordering System (An End-To-End LightSwitch Example)
Note: You must have Visual Studio Professional (or higher) to complete this tutorial
LightSwitch is a powerful application builder, but you have to get your data INTO it first. Usually this is easy, but in some cases, you need to use WCF RIA Services to get data into LightSwitch that is ALREADY IN LightSwitch.
LightSwitch operates on one Entity (table) at a time. A Custom Control will allow you to visualize data from two entities at the same time, but inside LightSwitch, each Entity is always separate. This can be a problem if you want to, for example, combine two Entities into one.
LightSwitch wont do that… unless you use a WCF RIA Service. Now you can TRY to use a PreProcess Query, but a PreProcess Query is only meant to filter records in the Entity it is a method for. You cannot add records, only filter them out (this may be easier to understand if you realize that a PreProcess Query simply tacks on an additional Linq statement, to “filter” the linq statement, that is about to be passed to the data source).
In this article, we will create a application that combines two separate entities and creates a single one. Note that this article is basically just a C# rehash of the Eric Erhardt article, but this one will move much slower, and provide a lot of pictures :). We will also cover updating records in the WCF RIA Service.
Also note, that the official LightSwitch documentation covers creating WCF RIA Services in LightSwitch in more detail: http://207.46.16.248/en-us/library/gg589479.aspx.
Create The Application
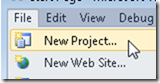
Create a new LightSwitch project called RIAProductInventory.
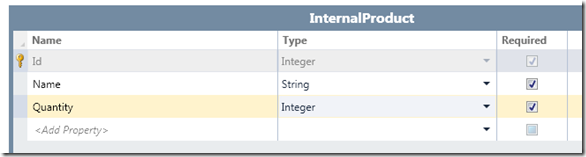
Make a Entity called InternalProduct, with the schema according to the image above.
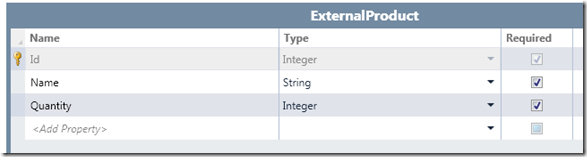
Make a Entity called ExternalProduct, with the schema according to the image above.
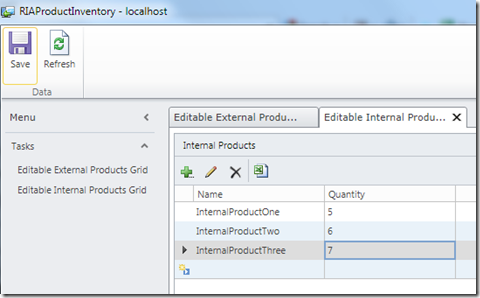
Create Editable Grid Screens for each Entity, run the application, and enter some sample data.
The WCF RIA Service
We will now create a WCF RIA Service to do the following things:
- Create a new Entity that combines the two Entities
- Connects to the existing LightSwitch database
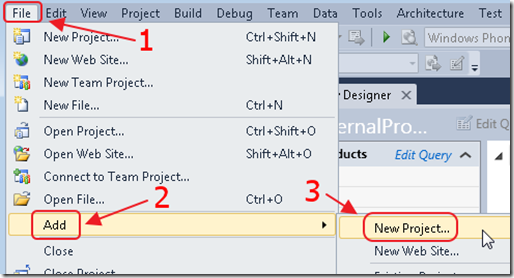
Create a New Project (Note: You must have Visual Studio Professional (or higher) to complete this tutorial).
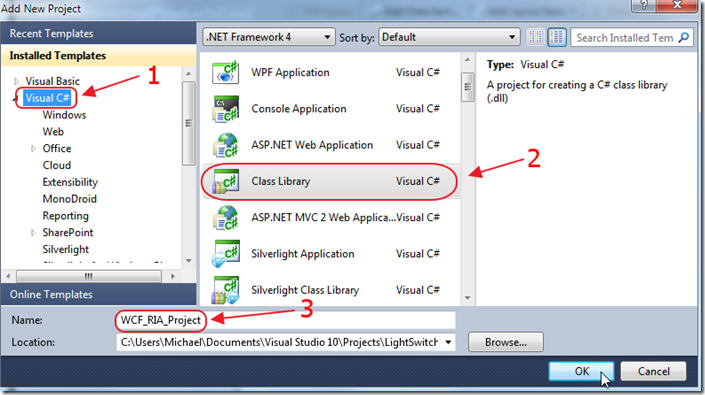
Create a Class Library called WCF_RIA_Project.
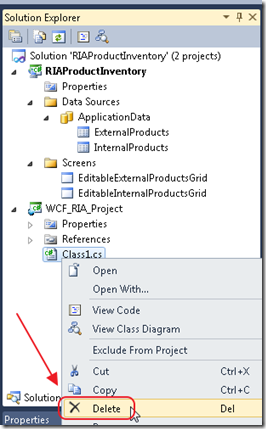
Delete the Class1.cs file that is automatically created.
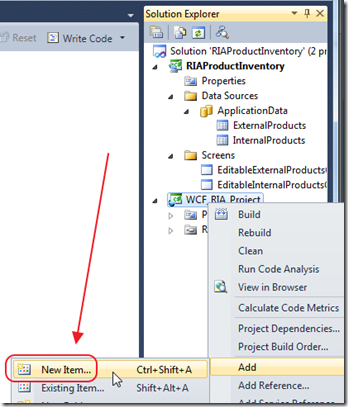
Add a New Item to WCF_RIA_Project.
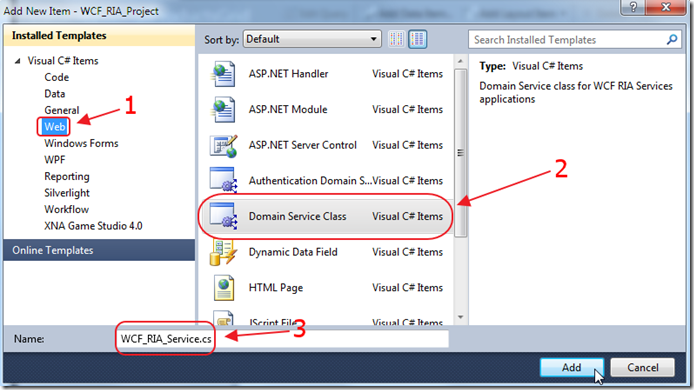
Add a Domain Service Class called WCF_RIA_Service.
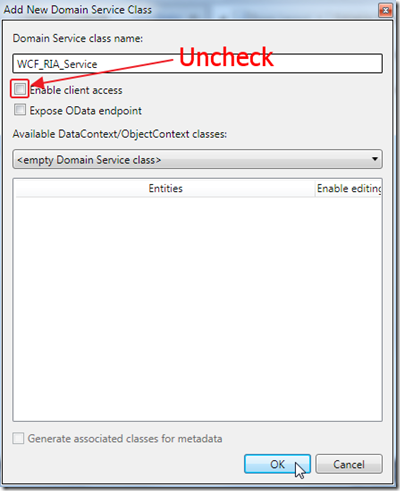
When the Add New Domain Service Class box comes up, uncheck Enable client access. Click OK.
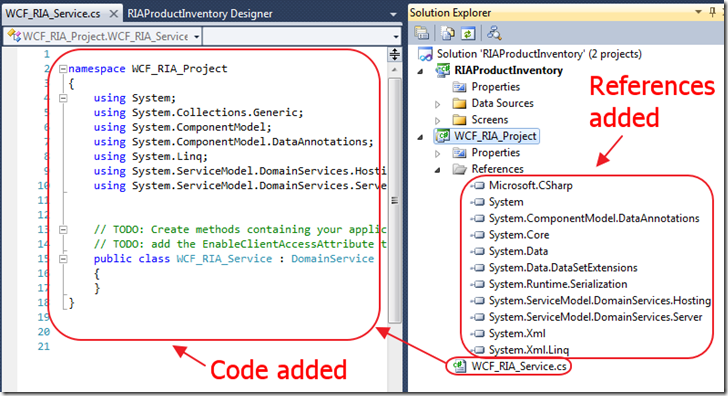
Code and references will be added.
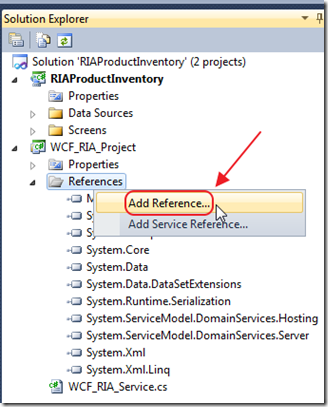
We need additional references, add the following references to the WCF_RIA_Project:
- System.ComponentModel.DataAnnotations
- System.Configuration
- System.Data.Entity
- System.Runtime.Serialization
- System.ServiceModel.DomainServices.Server (Look in %ProgramFiles%\Microsoft SDKs\RIA Services\v1.0\Libraries\Server if it isn’t under the .Net tab)
- System.Web
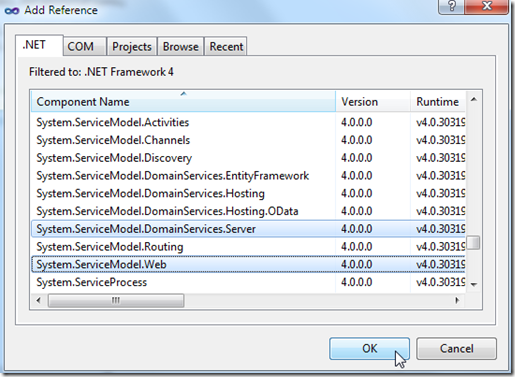
Add the following Using Statements to the class:
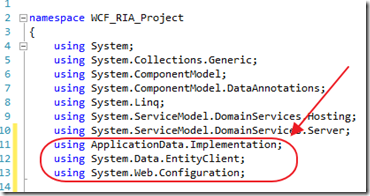
using ApplicationData.Implementation;
using System.Data.EntityClient;
using System.Web.Configuration;
Reference The LightSwitch Object Context
Now, we will add code from the LightSwitch project to our WCF RIA Service project. We will add a class that LightSwitch automatically creates, that connects to the database that LightSwitch uses.
We will use this class in our WCF RIA Service to communicate with the LightSwitch database.
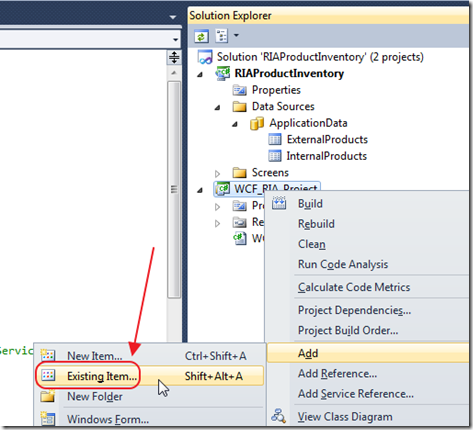
Right-click on the WCF_RIA_Project and select Add then Existing Item.
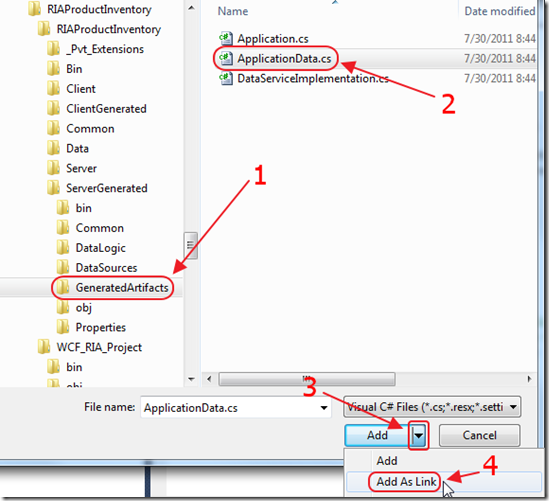
Navigate to ..ServerGenerated\GeneratedArtifacts (in the LightSwitch project)and click on ApplicationData.cs and Add As Link.
We used “add As Link” so that whenever LightSwitch updates this class, our WCF RIA Service is also updated. This is how our WCF RIA Service would be able to see any new Entities (tables) that were added, deleted, or changed.
Create the Domain Service
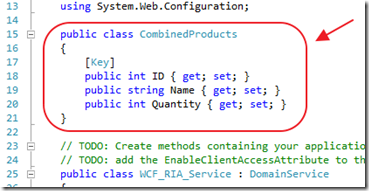
In the WCF_RIA_Service class, in the WCF RIA project, we now create a class to hold the combined tables.
Note, that we do not put the class inside the WCF_RIA_Service class. To do this would cause an error.
Use the following code:
public class CombinedProducts
{
[Key]
public int ID { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
}
Note that the “ID” field has been decorated with the “[Key]” attribute. WCF RIA Services requires a field to be unique. The [Key] attribute indicates that this will be the unique field.
Dynamically Set The Connection String
Next, Add the following code to the WCF_RIA_Service class:
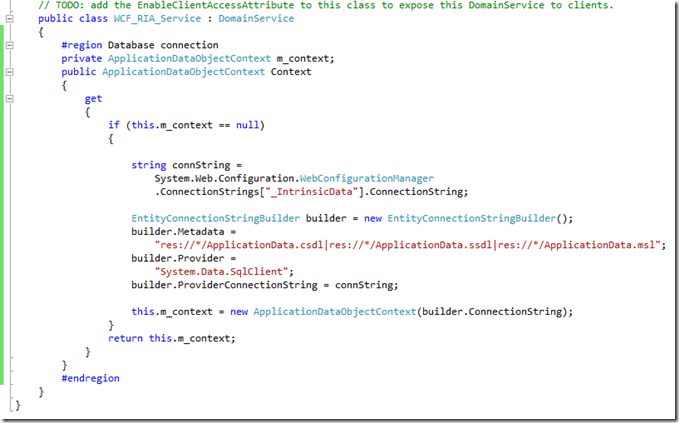
#region Database connection
private ApplicationDataObjectContext m_context;
public ApplicationDataObjectContext Context
{
get
{
if (this.m_context == null)
{
string connString =
System.Web.Configuration.WebConfigurationManager
.ConnectionStrings["_IntrinsicData"].ConnectionString;
EntityConnectionStringBuilder builder = new EntityConnectionStringBuilder();
builder.Metadata =
"res://*/ApplicationData.csdl|res://*/ApplicationData.ssdl|res://*/ApplicationData.msl";
builder.Provider =
"System.Data.SqlClient";
builder.ProviderConnectionString = connString;
this.m_context = new ApplicationDataObjectContext(builder.ConnectionString);
}
return this.m_context;
}
}
#endregion
This code dynamically creates a connection to the database. LightSwitch uses “_IntrinsicData” as it’s connection string. This code looks for that connection string and uses it for the WCF RIA Service.
Create The Select Method
Add the following code to the class:
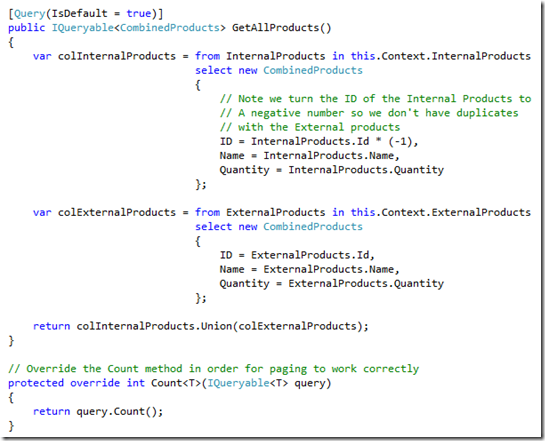
[Query(IsDefault = true)]
public IQueryable<CombinedProducts> GetAllProducts()
{
var colInternalProducts = from InternalProducts in this.Context.InternalProducts
select new CombinedProducts
{
// Note we turn the ID of the Internal Products to
// A negative number so we don't have duplicates
// with the External products
ID = InternalProducts.Id * (-1),
Name = InternalProducts.Name,
Quantity = InternalProducts.Quantity
};
var colExternalProducts = from ExternalProducts in this.Context.ExternalProducts
select new CombinedProducts
{
ID = ExternalProducts.Id,
Name = ExternalProducts.Name,
Quantity = ExternalProducts.Quantity
};
return colInternalProducts.Union(colExternalProducts);
}
// Override the Count method in order for paging to work correctly
protected override int Count<T>(IQueryable<T> query)
{
return query.Count();
}
This code combines the InternalProducts and the ExternalProducts, and returns one collection using the type CombinedProducts.
Note that this method returns IQueryable so that when it is called by LightSwitch, additional filters can be passed, and it will only return the records needed.
Note that the ID column needs to provide a unique key for each record. Because it is possible that the InternalProducts table and the ExternalProducts table can have records that have he same ID, we multiply the ID for the InternalProducts by –1 so that it will always produce a negative number and will never be a duplicate of the ExternalProducts Id’s that are always positive numbers.
Also notice that the GetAllProducts method is marked with the [Query(IsDefault = true)] attribute, one method, for each collection type returned, must not require a parameter, and be marked with this attribute, to be used with LightSwitch.
Consume The WCF RIA Service
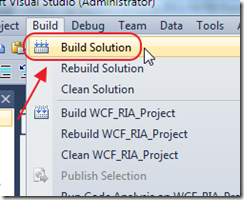
Build the solution.

You will get a ton or warnings. you can ignore them.
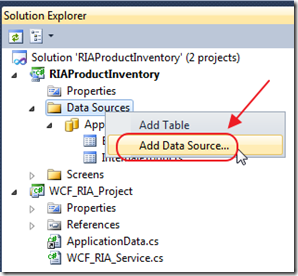
In the Solution Explorer, right-click on the Data Sources folder and select Add Data Source.
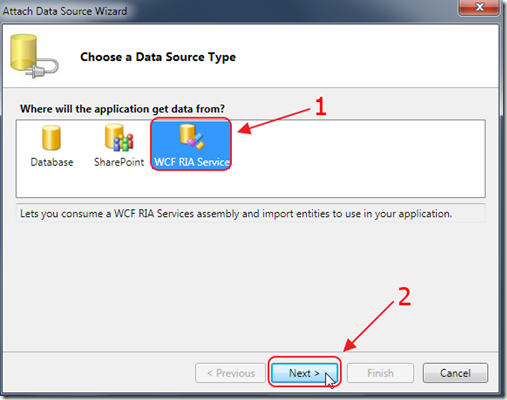
Select WCF RIA Service.
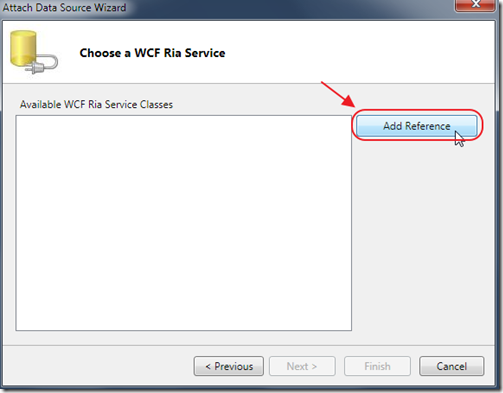
Click Add Reference.
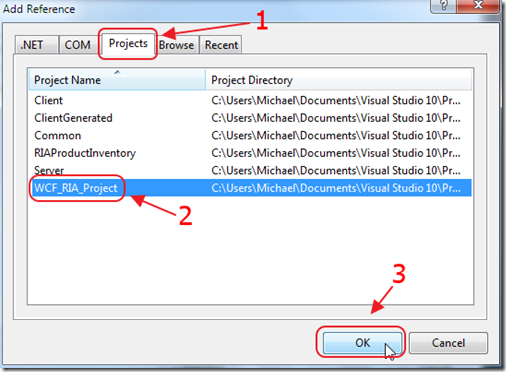
Select the RIA Service project.
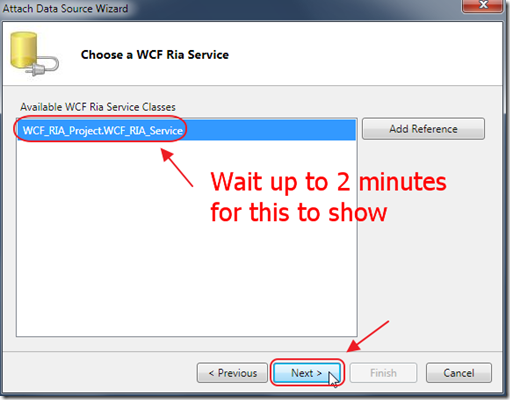
You have to wait for the service to show up in the selection box. Select it and click Next.
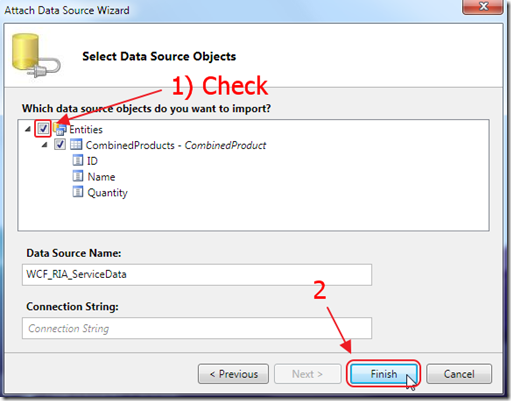
Check the box next to the Entity, and click Finish.
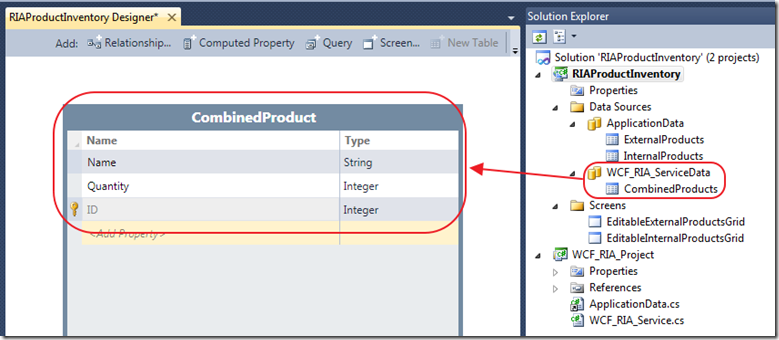
The Entity will show up.
Create a Screen That Shows The Combined Products
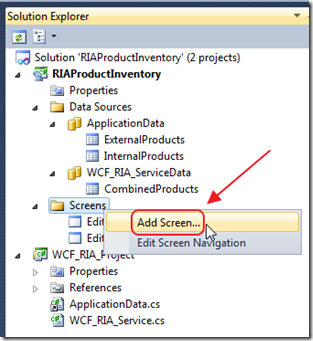
Add a Screen.
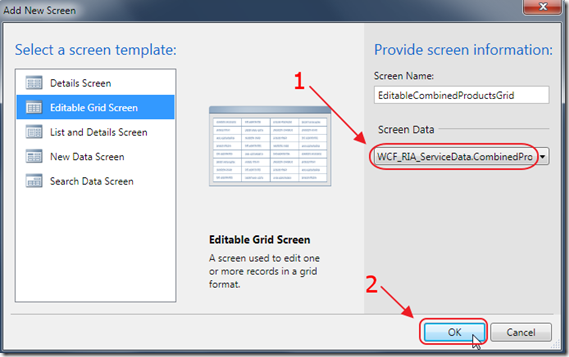
Add an Editable Grid Screen, and select the Entity for the Screen Data.
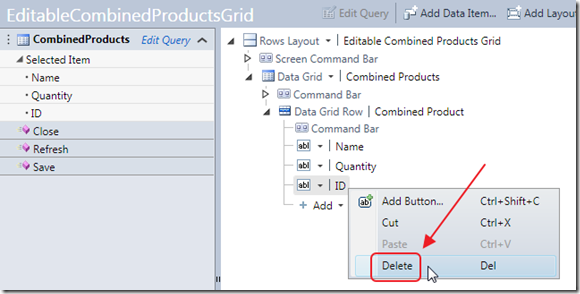
Delete the ID column (because it is not editable anyway).
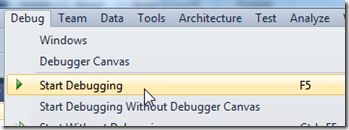
Run the application.
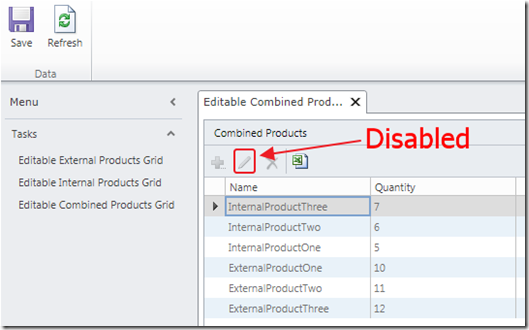
You will be able to see the combined Products.
However, you will not be able to edit them.
Updating Records In A WCF RIA Service
Add the following code to the WCF RIA Service
public void UpdateCombinedProducts(CombinedProducts objCombinedProducts)
{
// If the ID is a negative number it is an Internal Product
if (objCombinedProducts.ID < 0)
{
// Internal Product ID's were changed to negative numbers
// change the ID back to a positive number
int intID = (objCombinedProducts.ID * -1);
// Get the Internal Product
var colInternalProducts = (from InternalProducts in this.Context.InternalProducts
where InternalProducts.Id == intID
select InternalProducts).FirstOrDefault();
if (colInternalProducts != null)
{
// Update the Product
colInternalProducts.Name = objCombinedProducts.Name;
colInternalProducts.Quantity = objCombinedProducts.Quantity;
this.Context.SaveChanges();
}
}
else
{
// Get the External Product
var colExternalProducts = (from ExternalProducts in this.Context.ExternalProducts
where ExternalProducts.Id == objCombinedProducts.ID
select ExternalProducts).FirstOrDefault();
if (colExternalProducts != null)
{
// Update the Product
colExternalProducts.Name = objCombinedProducts.Name;
colExternalProducts.Quantity = objCombinedProducts.Quantity;
this.Context.SaveChanges();
}
}
}
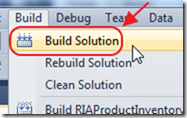
Build the solution.
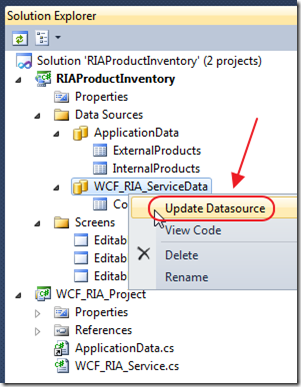
Right-click on the WCF_RIA_Service node in the Solution Explorer, and select Update Datasource.
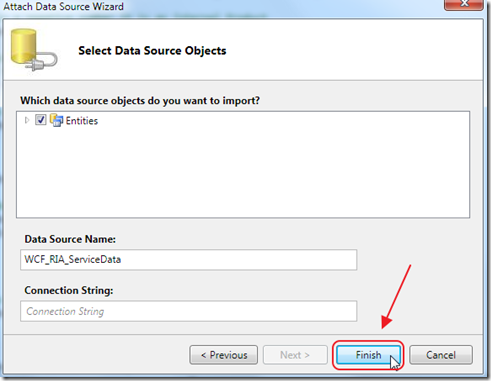
When the wizard shows up click Finish.
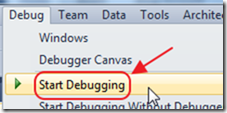
Run the application.
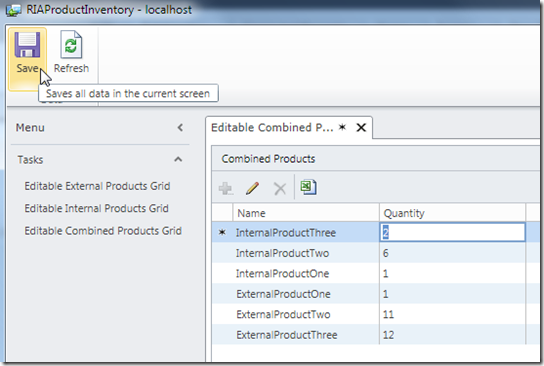
You will now be able to update the records.
Download Code
The LightSwitch project is available at http://lightswitchhelpwebsite.com/Downloads.aspx
77 comment(s) so far...
I always get a metadata exeption whenever the Context is being opened. The model does not seem to be found. There needs to be some magic to the csdl, ssl, msl files...
By Holger Flick on
8/3/2011 9:34 AM
|
@Holger Flick - download my sample and see if it works for you
By Michael Washington on
8/3/2011 10:24 AM
|
It works ... I see no difference to my project though only that your compiled dll resides in the same directory as the ls exe. Maybe that is the difference.
By Holger Flick on
8/3/2011 11:17 AM
|
@Holger Flick - open up a thread in the LightSwitch Hackers Forum (on this site) and if you can paste the code for your Domain Service.
Also indicate if you have checked: 1) You are using 'add as Link' 2) You are using released version of LightSwitch
By Michael Washington on
8/3/2011 11:32 AM
|
Great post. Couldn't believe how easy it was to add updating.
By Richard Waddell on
8/3/2011 5:16 PM
|
I'm wondering if this solution will also work for internationalized DBs. I have e.g. a product and a product_int table where the product table is the language and country independend part of the product and the product_int table contains the language specific part. Currently I'm hesitating using LightSwitch for an app with uses localized data in the UI and in the DB. I would strongly prefer to go the LightSwitch way but want to avoid to end in a dead end street.
By Uwe Helmut on
8/5/2011 4:44 AM
|
@Uwe Helmut - When you play around with LightSwitch more you will see that there is little worry of ending up in a "dead end street"
By Michael Washington on
8/5/2011 5:03 AM
|
I still cannot reproduce the article. I end up with uncompilable code in my LS app. Strangely enough if I select "Update datasource" two datasources show up. One datasource is named Projectname+Serviecname. The + character makes the generated code form LS fail. I have no idea where that comes from. LS only shows one datasource, but generates code for 2.
This is the code being generated that fails: protected override global::System.Type ConvertType(global::System.Type outerType) { if (outerType == typeof(global::ExternalRIAData.Implementation.BettingRoundProxy)) { return typeof(global::ExternalRIA.ExternalRIA+BettingRoundProxy); } return base.ConvertType(outerType); }
I also get 305 warnings that the entity types of my LS app are also found in the RIA service. I did enable client access so that I can access the same datasource from another app as well, which should not pose a problem.
By Holger Flick on
8/6/2011 12:09 AM
|
@Holger Flick - The problem you are having is the same problem I had when I first tried it. In my article please see the part where I say "do not put the class inside the WCF_RIA_Service class. To do this would cause an error." (there is a link to the forum post I made).
By Michael Washington on
8/6/2011 4:11 AM
|
Thanks a lot for the blog. It is impressive and very helpful with the boxes and arrows guiding. I like this style which will help the users unfamiliar to the software. It might be even better to illustrate the problem to be solved more visually and clearly at the beginning of the article.
By Joe Wu on
8/8/2011 4:50 AM
|
@Joe Wu - That is a good suggestion and I will keep it in mind in future articles.
By Michael Washington on
8/8/2011 4:51 AM
|
When I use wcf RIA when I use query.count () to paging,
protected override int Count (IQueryable query) { return query.Count (); }
query.Count() has value 55550 Data can not be loaded Tip unable to load data, please check your Network connection and try logding again But protected override int Count (IQueryable query) { return 50000; } Data can be loaded
By mysky on
8/16/2011 11:15 PM
|
@mysky - Please post technical questions to the forums: http://lightswitchhelpwebsite.com/Forum/tabid/63/aff/2/Default.aspx
By Michael Washington on
8/17/2011 4:10 AM
|
I had the same issue, if paging was enabled then data cannot be loaded. Then I started up the Distributed Transaction Coordinator service (MSDTC) on the database server. After this, the paging problem was solved.
By Danny on
8/30/2011 3:03 PM
|
Please see this article: http://blogs.msdn.com/b/mthalman/archive/2010/09/20/how-to-reference-security-entities-in-lightswitch.aspx
about using:
"Transaction.Current = null;"
"to avoid the need for a distributed transaction"
By Michael Washington on
8/30/2011 3:06 PM
|
Example works well when creating Entities from within Lightswitch, however when creating a similar example using an external database the Count method:
// Override the Count method in order for paging to work correctly protected override int Count(IQueryable query) { return query.Count(); }
always fails with a:
"An error occurred while executing the command definition. See the inner exception for details."
The inner exception is:
"Invalid object name 'dbo.GameData'."
GameData is one of my external SQL tables mapped to a Lightswitch Entity (both are named GameData). Any ideas here?
By Todd on
9/21/2011 3:51 PM
|
@Todd - in LightSwitch is is probably not called dbo.GameData" but GameData" or "GameDataSet". Also post technical questions to the forums. This comments section is lousy for that sort of thing.
By Michael Washington on
9/21/2011 4:09 PM
|
@Todd,
Hi Todd,
Did you find a solution for problem? I've got the same issue.
Thanks,
Daniel
By Daniel on
9/29/2011 11:26 AM
|
Thanks for the blog Michael,
I was able to get my app working following your steps but When I publish the app. The screen that I created is just showing Blank and I also couldn't find entity created by RIA in the published DB.
I guess it has something to do with "Other Connections" screen in Publish Application wizard.
could you please put some light on how to publish a Lightswitch App when using WCF RIA services?
Thanks already.
Regards, Akhil
By Akhil on
10/1/2011 4:09 AM
|
@Akhil - When using this method there is only one database connection. The Publish process should be normal.
By Michael Washington on
10/1/2011 4:11 AM
|
Great article.
I changed the m_context declaration to:
private static ShotEntryDataObjectContext m_context;
This works and does not creates a new DataObjectContext each time the Context prpoerty is called.
Can you think of any negative impact from this?
Thanks, Dave
By fishy on
10/2/2011 7:19 AM
|
@fishy - I can think of no negative impact. Let us know how it works for you.
By Michael Washington on
10/2/2011 7:47 AM
|
Thanks for this tutorial, Michael. I'm a vb person. Should I be able to reproduce tutorial this in vb, with the obvious language changes? I get as far as loading the vb equivalent to ApplicationData.cs then get stuck. I see 3 possible vb equivalents to this file but none of them give me the vb equivalent to the cs "Using..." statements that are needed. I'm a long time Access/VBA programmer and am still climbing up the learning curve for Visual Studio. Should the references and "Using" statements ("Imports" in vb) be the same?
Thanks, E.
By emanning on
10/4/2011 5:29 AM
|
Ack! That should be "Should I be able to reproduce this tutorial in vb..."
By emanning on
10/4/2011 5:31 AM
|
@emanning - Yes you should be able to reproduce it in VB. However, unfortunately I am slammed with projects and unable to have the time. I am sorry.
By Michael Washington on
10/4/2011 7:11 AM
|
"you should be able to reproduce it in VB" That's all I needed to know. Thanks. I'll learn more doing it on my own and using your example for help.
By emanning on
10/4/2011 9:20 AM
|
Hi Michael, I am about to do this tutorial. But you might be able to save me some time. I am trying to get the profile data from the dotnetnuke userprofile joined with the profilepropertydefinitions tables. Frankly I just need to grab the related phone numbers. My lightswitch project is desired due to its Office Integration capabilities. Anyway, do you know of an efficient way to get that profile data from the dotnetnuke tables? Joe Brinkman wrote an excellent query, but I can't make it work with Linq and Lightswitch, it is Un-IQueryable.
By wdarnellg on
10/11/2011 7:11 AM
|
@wdarnellg - I don't have anything, but make a post to the forums on this site.
By Michael Washington on
10/11/2011 8:27 AM
|
Hi Machael,
First of all, thank you for yet another useful article. I've implemented a service like the one you describe but I can't get the update method to work. My data is read-only when I display them on the LS screen. I've done a few things differently that shouldn't have any impact, but then again...
- The GetAll() method always returns an empty set - I've created another GetWithParam method that does return data, and this is the only method in use - When building instances of the entity, I use a sub-select to another table to set some properties - I am using an externally attached database
Do you have any ideas what could cause this?
All the best, Rune
By Rune on
11/24/2011 7:54 AM
|
@Rune - Please post any technical questions to the forums. Also there is a format code button to paste any code.
By Michael Washington on
11/24/2011 7:55 AM
|
Any ideas how to get rid of the warnings? The solution works, but I get over 1000 warnings. I know they are ignorable, but what if I produce some warnings myself that I should fix. Leaves me with some bad feeling...
So I took another approach: I add three assembly references to my class library: Microsoft.LightSwitch.dll, Application.Common.dll and Application.ServerGenerated.dll. The last two can be found under Project\ServerGenerated\bin. The first assembly is located under the many Debug/Release folders (it should be make any difference, which one to use). Don't know how well this works, wenn the Server side code gets updated, because it is the least one in the Project Dependencies and therefore I don't get the updated dll the first time I compile the Project.
By Tobias on
12/14/2011 5:55 AM
|
Hi thanks for your helpfull article, in my WCF_RIA_Service.cs I get an error at line using ApplicationData.Implementation; Error 2 Type- or namespace "ApplicationData" could not be found.
I made sure that all the references specified are added to my project.
Appreciate any help Thanks Bodo
By Bodo on
1/14/2012 6:37 AM
|
@Bodo - Please download the code from the download page and compare it to your own.
By Michael Washington on
1/14/2012 6:38 AM
|
@Bodo - I had the same issue in that it did not 'like' "using ApplicationData.Implementation;" However, I found that if you just comment it out and carry on with this guide you will get to a part where "ApplicationDataObjectContext" requests that "using". If you add it at this point it will work fine.
I don't know why it does this... but this is a way around it.
By Craig on
2/2/2012 3:14 AM
|
Thanks for the article Michael, I don't need to combine tables and don't need updates. I need this to reduce number of columns to fix the search. In Lightswitch search will search all the columns in the data source even if they are not shown. If query(request) sent to the server exceeds 2000+ characters it would show an obscure error. As described I added the library project, domainservice, linked context. It worked. Then I tried to add the domainservicer directly under the server "usercode" (file view). I was not able to use the context so I added the Linq to SQl context and it worked. No warnings. Deplyed it without a glitch.
By AlexK on
2/6/2012 6:30 PM
|
Michael, great article! Thank you. How would I modify the code to connect a web service to a table? I've modified code from powerbala (http://powerbala.com/2011/04/16/calling-a-3rd-web-service-from-lightswitch/) to connect to a 3rd Party Web Service in order to get data, but can't filter the web service data using data in an internal table. Thanks again for your expertise.
By brian norberg on
2/15/2012 1:12 PM
|
@brian norberg - I am sure it is possible but I have no code samples, sorry :(
By Michael Washington on
2/15/2012 2:47 PM
|
is there a website that would convert the C# code to Vb? OR is there a way to turn my exisiting VB lightswitch application into C# without having to start all over again?
I am starting with VB but if C# is better, I would like to go that route if I can convert my project into C# without having to start from the beginning.
By dbdmora on
2/27/2012 6:20 PM
|
Hi Michael,
if I need a field is a list ?
Thanks.
By Niko on
5/8/2012 8:23 AM
|
And for those playing with LightSwitch in Visual Studio 2012 RC. Remember to set the target framework in your WCF RIA Service to .NET framework 4 (default is 4.5).
Nice article Michael, thanks.
By Ralf de Kleine on
6/12/2012 12:36 PM
|
And also a good tip add multiple workspaces to prevent readonly controls.
Private Sub CustomersListDetail_InitializeDataWorkspace( saveChangesTo As System.Collections.Generic.List(Of Microsoft.LightSwitch.IDataService))
saveChangesTo.Add(Me.DataWorkspace.ApplicationData) saveChangesTo.Add(Me.DataWorkspace.Team_SiteData) End Sub
Link to Beth Massi's post: http://blogs.msdn.com/b/bethmassi/archive/2011/07/11/relating-and-editing-data-from-multiple-data-sources-on-the-same-screen.aspx
By Ralf de Kleine on
8/21/2012 12:15 PM
|
I've check and rechecked my code but I get an error:
Error 16 The type name 'DetailsClass' does not exist in the type 'LightSwitchApplication.statusReportReviewTabView'
I only get this when I add the WCF RIA service into my lightswitch application. Both projects compile fine when my service is not added to the lightswitch app.
I renamed the table you had defined to statusReportReviewTabView for a project I'm working on.
I'm using Visual Studio 2012.. Any ideas?
By Kyle on
8/21/2012 12:14 PM
|
@Kyle - Download the code and compare it to your own. Also see this article for changes needed to make it work with LightSwitch 2012 (article says LightSwitch 11 because the name wasn't finalized yet): http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/109/Using-WCF-RIA-Services-With-LightSwitch-11.aspx
By Michael Washington on
8/21/2012 12:18 PM
|
Well.. my code looked ok. So I created a new project in 2012 (the old 2012 project had been converted from 2010) and put my code into it. It worked fine. I'll keep trying to find out why the converted 2010 project wasn't working. I may have added my references in before I switched it from .net 4.5 to 4.0. Maybe this is the cause.
By Kyle on
8/23/2012 3:23 PM
|
Hi,
I am drafting the layouts of an application. De basic "administrative" application would be written in Lightswitch, but the data of the entities should also be used by a WPF dektop application in production enviroment with touch interface. This WPF application is mostly UI and all Business logic should be in the entities of lightswitch. The WPF application schould not process Odata, but just makes WCF calls to GET related XML data from the lightswitch via function calls with parameters and returns data and SET data via function calls also, sending XML or other parameters als input parameters of the function.
I was thinking of writing a WCF Ria service that connects to the Lightswitch enities and do all the work in functions that can be called via WCF from the WPF application.
Any feedback or links where I could find more info would be nice !
Thanks Danny
PS Great blog, great info, Keep up the good work !
By Danny on
10/17/2012 2:04 PM
|
@Danny - All the articles on this site on WCF RIA Services is at: http://lightswitchhelpwebsite.com/Blog/tabid/61/tagid/21/WCF-RIA-Service.aspx
By Michael Washington on
10/17/2012 2:05 PM
|
I have made the WCF RIA Services using the above post. But when I Add this as the datasource and run the solution, it gives me the following error.
1. ) expected
2. Invalid expression term ')'
3. ; expected
4. A new expression requires (), [], or {} after type
The above error are generated in the following class..
..\ServerGenerated\GeneratedArtifacts\DataServiceImplementation.cs
Any help will be appreciated..
Best,
Inayat Rehman
By Inayat Rehman on
1/9/2013 5:18 AM
|
********************************** Please post any technical questions to the forums - The Blog comments are not a good place to answer technical questions. Any technical questions posted will not be answered - Sorry **********************************
By Michael Washington on
1/9/2013 5:20 AM
|
Thanks for a great article I have it working with two internal tables and with a SOAP Web Service. That must be good for someone who is new to Silverlight, Lightswitch and WCF RIA. My only concern is what about Add and Delete I see how the update works but no mention Add or Delete?
Great Article Thanks Again
By MisterB on
3/15/2013 2:14 AM
|
In Visual Studio 2012 Update 2 (or higher) some of the steps have changed. See: Creating a WCF RIA Service for Visual Studio 2012 (Update 2 and higher) http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/1194/Creating-a-WCF-RIA-Service-for-Visual-Studio-2012-Update-2-and-higher.aspx
By Michael Washington on
5/4/2013 10:36 AM
|
For the update/insert/delete methods to work, also make sure that your Save Data Source is set to your Ria Service. LS does this automatically when creating a screen for an entity of that data source, but you can easily miss it if you are refactoring.
By Chielus on
7/22/2013 11:40 PM
|
Or, in case of multiple editable data sources, you can expand the saveChangesTo object as shown in http://msdn.microsoft.com/en-us/vstudio/hh313625.aspx
By Chielus on
7/23/2013 12:01 AM
|
@Chielus - Thank You
By Michael Washington on
7/23/2013 4:32 AM
|
Hello Michael, I'm really learning from your website.. helped me a lot :) Thanks..
I'm really stuck at (what it seems) a very -very- simple project, with LS and RIA services.. It is explained in details here: http://social.msdn.microsoft.com/Forums/vstudio/en-US/f7949b81-e941-4b9b-80c4-aa06d77c4436/unable-to-cast-error-in-lightswitch-runtime-wcf-ria?prof=required
Can you please help me with it? Thanks in advance for your help..
By walrashoud on
7/23/2013 3:10 PM
|
I download your source code running under VisualStudio 2012 Dotnet Framework 4.0, unfortunately got this error at line 74 of AppliationData.cs. The error message said:" InvadidOperationException was unhandled by user code" detail error:{"Mapping and metadata information could not be found for EntityType 'ApplicationData.Implementation.InternalProduct'."}
By Steven on
7/25/2013 5:51 PM
|
@Steven - See Fixing WCF RIA Services After Upgrading To HTML Client (http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/164/Fixing-WCF-RIA-Services-After-Upgrading-To-HTML-Client.aspx) and Creating a WCF RIA Service for Visual Studio 2012 (Update 2 and higher) (http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/1194/Creating-a-WCF-RIA-Service-for-Visual-Studio-2012-Update-2-and-higher.aspx)
By Michael Washington on
7/25/2013 6:04 PM
|
It is probably a stupid question, but is it working for Desktop Lightswitch App ?
Because i did exactly what you explain on your tutorial and when i debug the WCF function, it seems that the connection to the Data can't be done correctly.
I also tried to create the connection directly using : _connectionString = "data source=NorthwindDB;initial catalog= " + "Northwind;user id=myID;password=myPassword";
but same result.
Any ideas ? And thanks for your help in those tutorials !
By Clem on
8/21/2013 8:09 AM
|
@Clem - It should work with a desktop application as is, but I have not tested it, sorry :(
By Michael Washington on
8/21/2013 8:11 AM
|
I cant find "Domain Service Class" in Visual Studio 2013, what can i do?
By NESTicle on
9/25/2013 11:06 AM
|
@NESTicle - For Visual Studio 2013 you want to use: http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/2226/Creating-a-WCF-RIA-Service-for-Visual-Studio-2013.aspx
By Michael Washington on
6/17/2014 5:01 AM
|
Hi Michel, On HTML Client, Is it possible to make the Update part if I'm not using all fields on the combined entity?
Thanks.
By Fernando on
12/17/2013 5:59 AM
|
@Fernando - Yes. You may find the examples at this link helpful: http://lightswitchhelpwebsite.com/Blog/tabid/61/tagid/21/WCF-RIA-Service.aspx
By Michael Washington on
12/17/2013 6:23 AM
|
Great article. Really helpful and well explained. I have tried it and worked correctly.
Thank you,
Vassilis
By Vassilis Tsolekas on
6/17/2014 4:59 AM
|
How would you add multiple items via RIA, would you make a separate .cs for each entity you want to see in LS or do you make a whole new WCF RIa project?
By John on
9/26/2014 3:45 AM
|
@John - One method can handle inserting records to multiple tables. See: http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/2227/LightSwitch-Survey-Handling-Complex-Business-Logic-Using-WCF-RIA-Services.aspx
By Michael Washington on
9/26/2014 4:01 AM
|
I've modified this example to use an added Data Source that points to my company's ERP system. I have all the configuration correct in the RIA service class and am querying the database with LINQ. I'm creating a list though with several different queries and then returning my list of new objects AsQueryable. When I debug the service I see that everything I expect to be in the list, but when it renders on a Browse Data screen in LightSwitch HTML, it only shows the first record in the list over and over (actually the total count of the entities in the query). I also turned off paging to make sure that has nothing to do with it.
I think that it's something on the LightSwitch side as the service is making the correct list. Any ideas on this one?
By cmsmith on
10/16/2014 1:40 PM
|
@cmsmith - You should be able to set a break point and debug the service to see exactly what is going on.
By Michael Washington on
10/16/2014 2:18 PM
|
I do not have ApplicationData.cs file. I am using VS 2013 then what file to link
By mahendra on
12/9/2014 1:25 PM
|
@mahendra - Please see the VS2013 version here: http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/2226/Creating-a-WCF-RIA-Service-for-Visual-Studio-2013.aspx
By Michael Washington on
12/9/2014 1:27 PM
|
Unable to load data from entities. I am using VS 2013
By mahendra on
12/9/2014 3:32 PM
|
@mahendra - This sample was not tested in VS2013, sorry.
By Michael Washington on
12/9/2014 3:48 PM
|
Now, I am able to configure the environment successfully but Unable to load data from entities. I am using VS 2013
By mannu on
12/9/2014 4:25 PM
|
@mahendra - Please post to the official LightSwitch forums for assistance: https://social.msdn.microsoft.com/Forums/vstudio/en-US/home?forum=lightswitch
By Michael Washington on
12/9/2014 4:39 PM
|
Michael, please accept our small developer team appreciation. It worked perfectly and has been most useful.
Respectueusement, Denis
By covijt on
1/11/2015 1:40 PM
|
@covijt - Thank You :)
By Michael Washington on
1/11/2015 3:04 PM
|
Domain Service Class is missing in my visual studio 2015,,how can i get it??
By Mahoda on
7/11/2016 2:54 PM
|
@Mahoda - See "Creating a WCF RIA Service for Visual Studio LightSwitch 2013": http://lightswitchhelpwebsite.com/Blog/tabid/61/EntryId/2226/Creating-a-WCF-RIA-Service-for-Visual-Studio-2013.aspx
By Michael Washington on
7/11/2016 2:57 PM
|