Apr
11
Written by:
Michael Washington
4/11/2011 5:49 AM
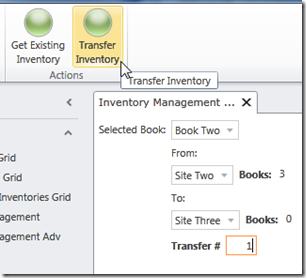
LightSwitch is designed for “forms over data”, entering data into tables, and retrieving that data. Procedural code is required when you need to manipulate data in “batches”. Normally, you want to put all your custom code on the Entity (table) level, but for procedural code, you want to use custom code on the Screen level.
The Inventory Program
To demonstrate procedural code in LightSwitch, we will consider a simple inventory management requirement. We want to specify sites and books, and we want to move those books, in batches, between the sites.
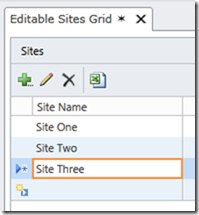
We enter sites into a table.
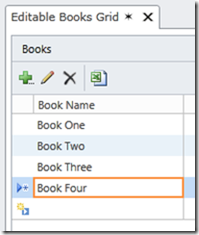
Each book is entered into the database.
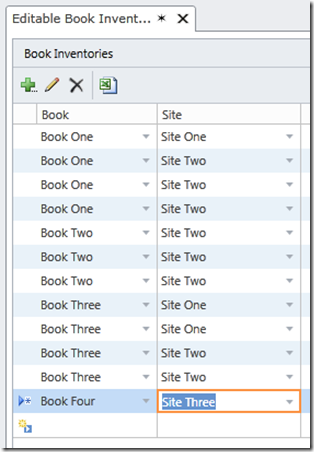
We create one record for each book, and assign it to a site (yes a better interface to do this could be created using the techniques explained later).
Inventory Management (non-programming version)
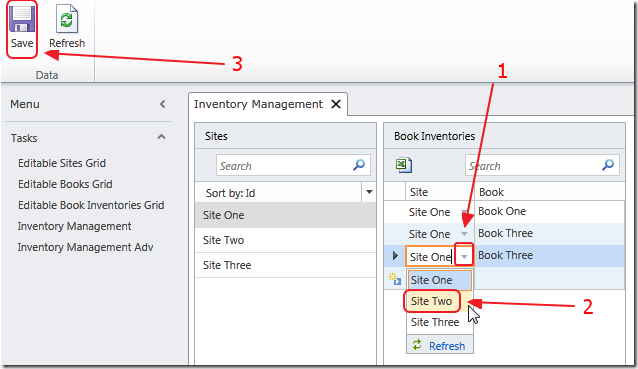
Even procedural programming is simply moving data around. If you are not a programmer, you can simply make a screen that allows a user to change the site for each book. The problem with this approach is that it would be time-consuming to transfer a large amounts of books.
Inventory Management (using programming)
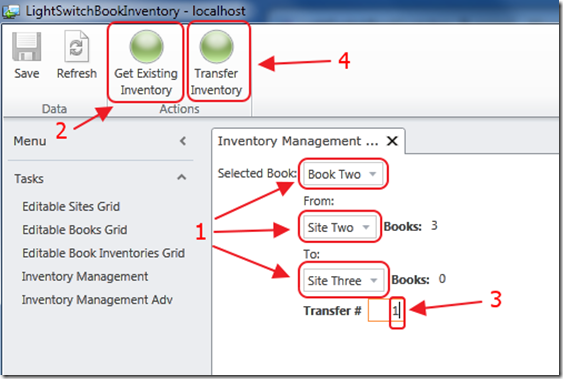
Using a little programming, we can create a screen that allows us to update the book locations in batches.
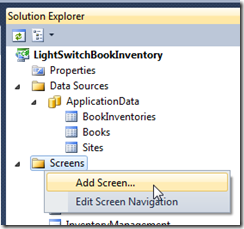
First we add a Screen.
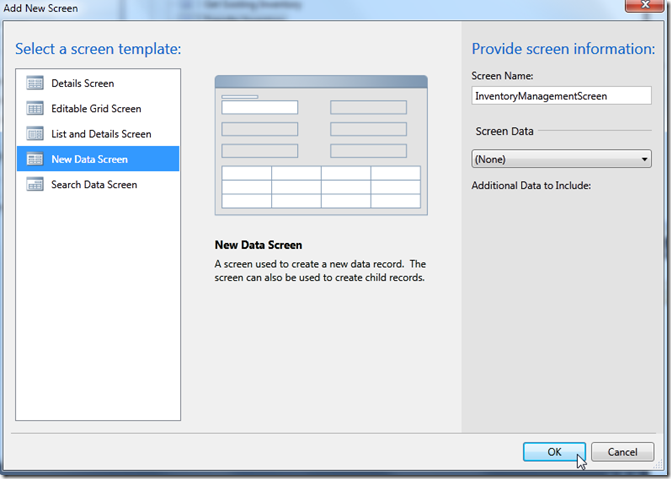
We give the Screen a name, we select New Data Screen, and we do not select any Screen Data.
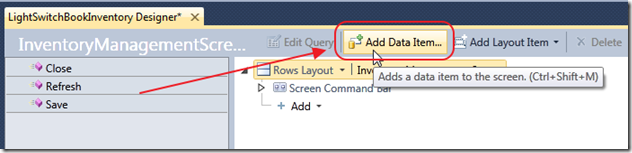
We Add Data Item.
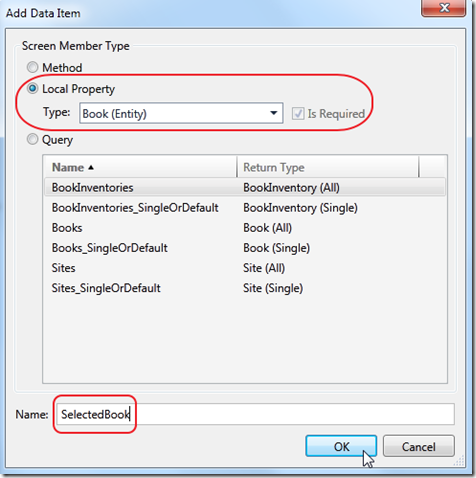
We add a Property that will allow us to select a book.
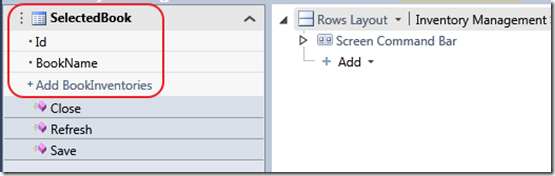
It will show in the Screen designer.
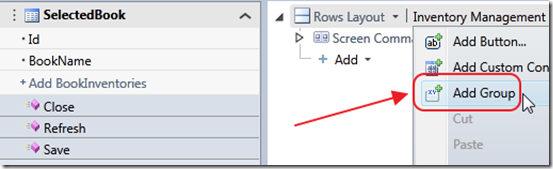
We right-click on the top group, and select Add Group.
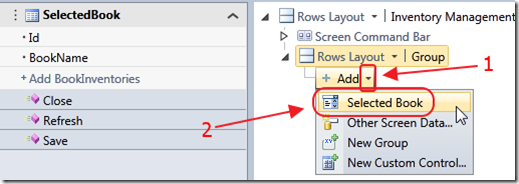
We are then able to add Selected Book to the screen as a drop down.
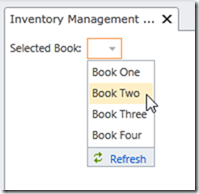
If we run the application at this point, we see that it allows us to select a book.
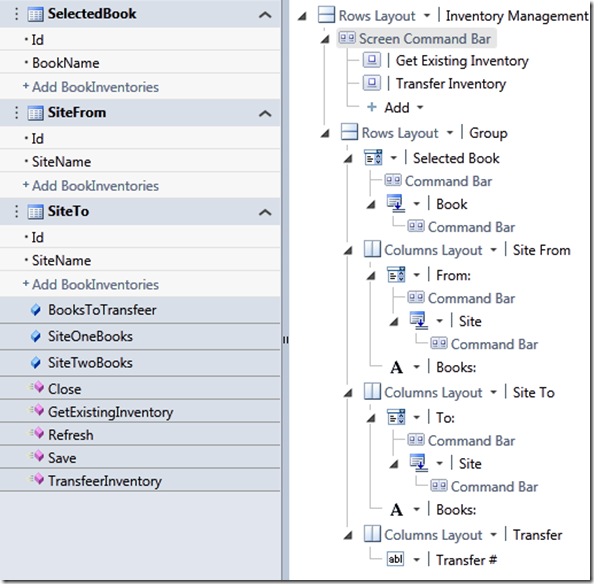
We add the various elements to the Screen.
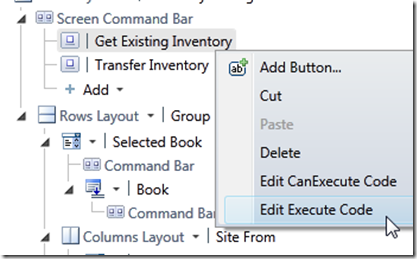
Here is the code we use for the Get Existing Inventory button:
partial void GetExistingInventory_Execute()
{
if (SiteFrom != null)
{
SiteOneBooks = SiteFrom.BookInventories.Where(x => x.Book == SelectedBook).Count();
}
if (SiteTo != null)
{
SiteTwoBooks = SiteTo.BookInventories.Where(x => x.Book == SelectedBook).Count();
}
}
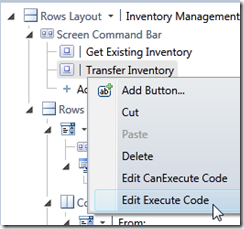
Here is the code for the Transfer Inventory button:
partial void TransfeerInventory_Execute()
{
// Ensure that we have all needed values
if ((SiteFrom != null) && (SiteTo != null) && (BooksToTransfeer > 0))
{
// Get the Books from SiteFrom
// Loop based on the value entered in BooksToTransfeer
for (int i = 0; i < BooksToTransfeer; i++)
{
// Get a Book from SiteFrom that matches the selected Book
var BookFromSiteOne = (from objBook in SiteFrom.BookInventories
where objBook.Book.BookName == SelectedBook.BookName
select objBook).FirstOrDefault();
// If we found a book at SiteFrom
if (BookFromSiteOne != null)
{
// Transfeer Book to SiteTo
BookFromSiteOne.Site = SiteTo;
}
}
// Save changes on the ApplicationData data source
this.DataWorkspace.ApplicationData.SaveChanges();
// Set BooksToTransfeer to 0
BooksToTransfeer = 0;
// Refresh values
GetExistingInventory_Execute();
}
}
The download for the complete project is here:
http://silverlight.adefwebserver.com/files/LightSwitch/LightSwitchBookInventory.zip
1 comment(s) so far...
Very good. Nice example of how to do some procedural code, and working through the screen designer with the download open really expanded my understanding of how to put a screen together with the controls and data of my choosing.
By Richard Waddell on
4/14/2011 6:32 PM
|